Globum Nix
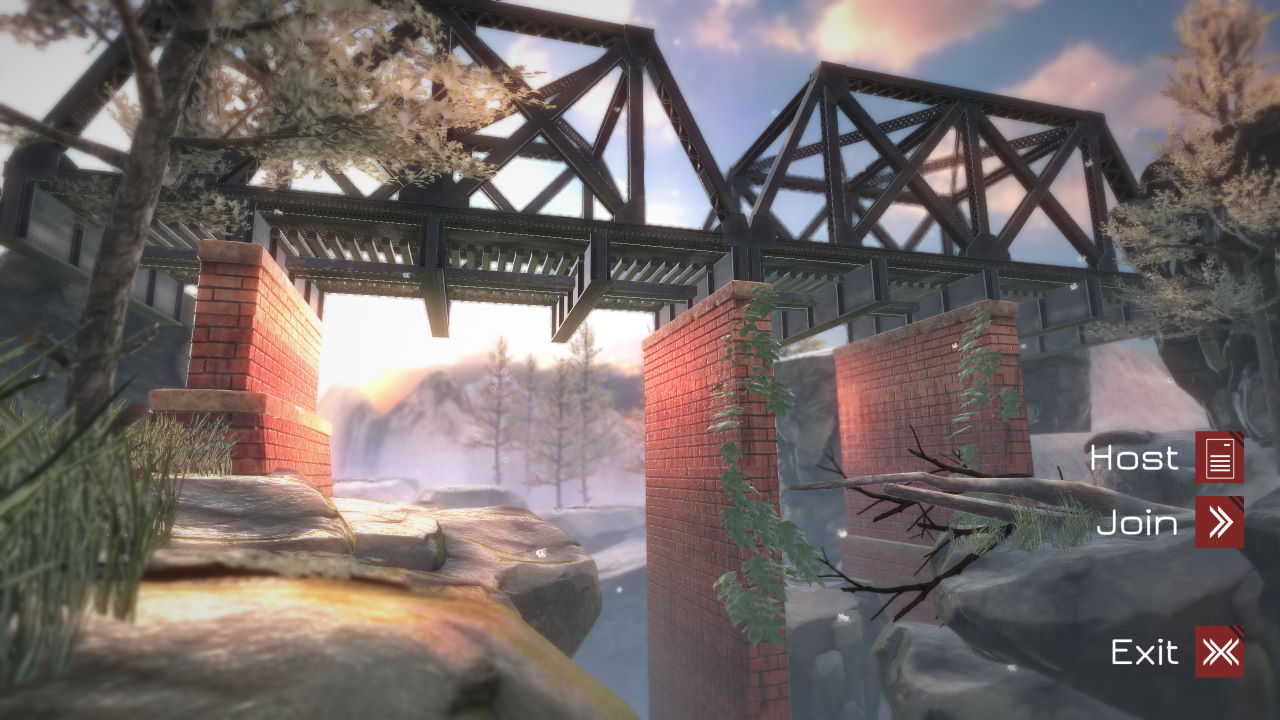
Globum Nix is an online-multiplayer first-person, team-based snowball fighting game. Created in Unity 5.0.
My contributions
- Networking middleware. I implemented the networked logic and synchronization protocol on top of Lidgren. It provides the ability to synchronize networked
GameObject
s and their associatedNetBehaviour
s. ANetBehaviour
can perform networked synchronization via two methods, either by invokingNetMethod
s (single or multicast RPCs), or by marking fields with[SyncVar]
. Below is an example:
class Health : NetBehaviour
{
// Reliable SyncVars are only transmitted on change and sent reliably.
// Unreliable SyncVars are continuously transmitted but individual,
// updates may be missed.
[SyncVar(SendMethod.Reliable)]
private int _health;
private NetMethod<Action<Vector3>> _spawnParticle;
protected internal override void NetAwake()
{
_spawnParticle = NetMethod.Create<Vector3>(position);
_health = 100;
}
public void OnTakeDamage()
{
// Asserts that this is running on the server
NetContext.Server();
// [SyncVar] takes care of the synchronization
_health -= 1;
// RPC that runs on all clients, with a single parameter
_spawnParticle.OnClients()(transform.position);
}
[NetMethod(SendMethod.Reliable)]
private void SpawnParticle(Vector3 position)
{
// Asserts that this runs on clients only
NetContext.Client();
// ...
}
}
- DX11 compute shader particle simuation for the snowfall. It uses the heightmap of the terrain to adjust the trajectory of the snow particles. The video demonstrates the effect, however the number of particles has been tuned down, so that it's better visible with video compression:
- DX11 tesselation based terrain with realtime and persistent deformation. The terrain converted from Unity's built-in terrain to allow the placement tools for other assets to be used. The system synchronizes deformation across the network, and only holds them in VRAM for nearby chunks of the terrain. Its purpose is to show footprints and where other players grabbed snow. The screenshots below show a spot before and after a deformation:
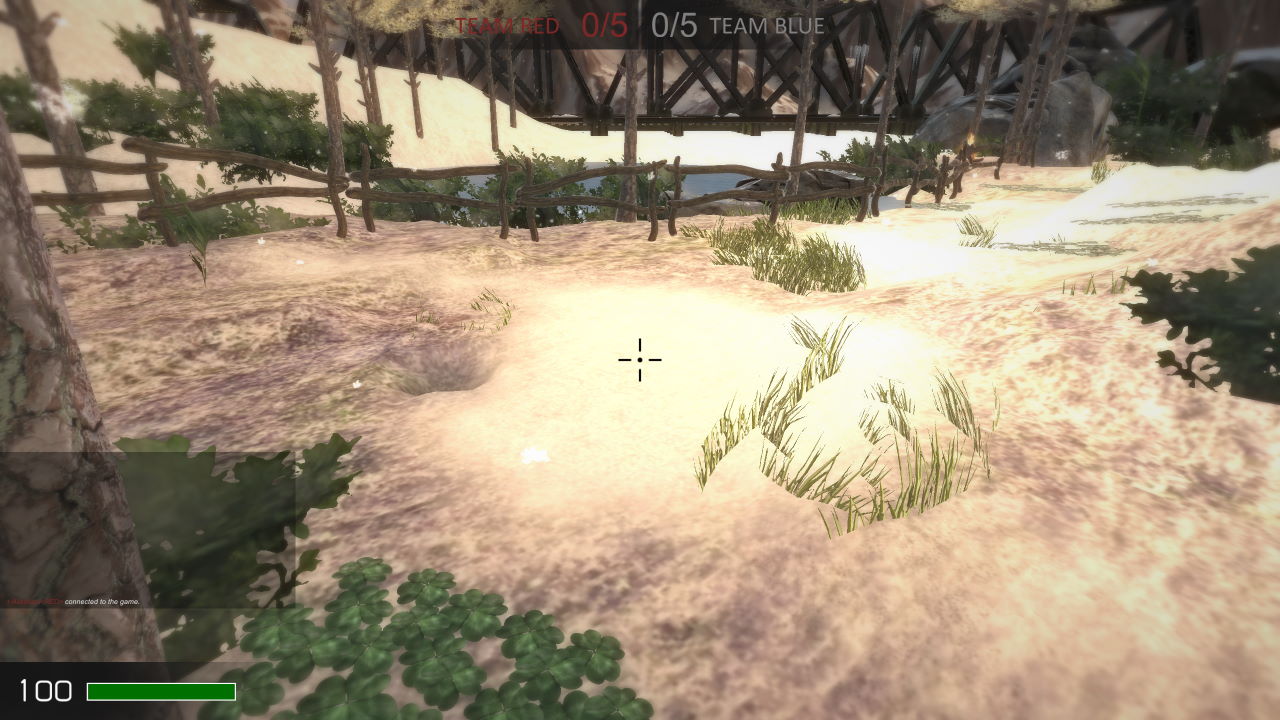
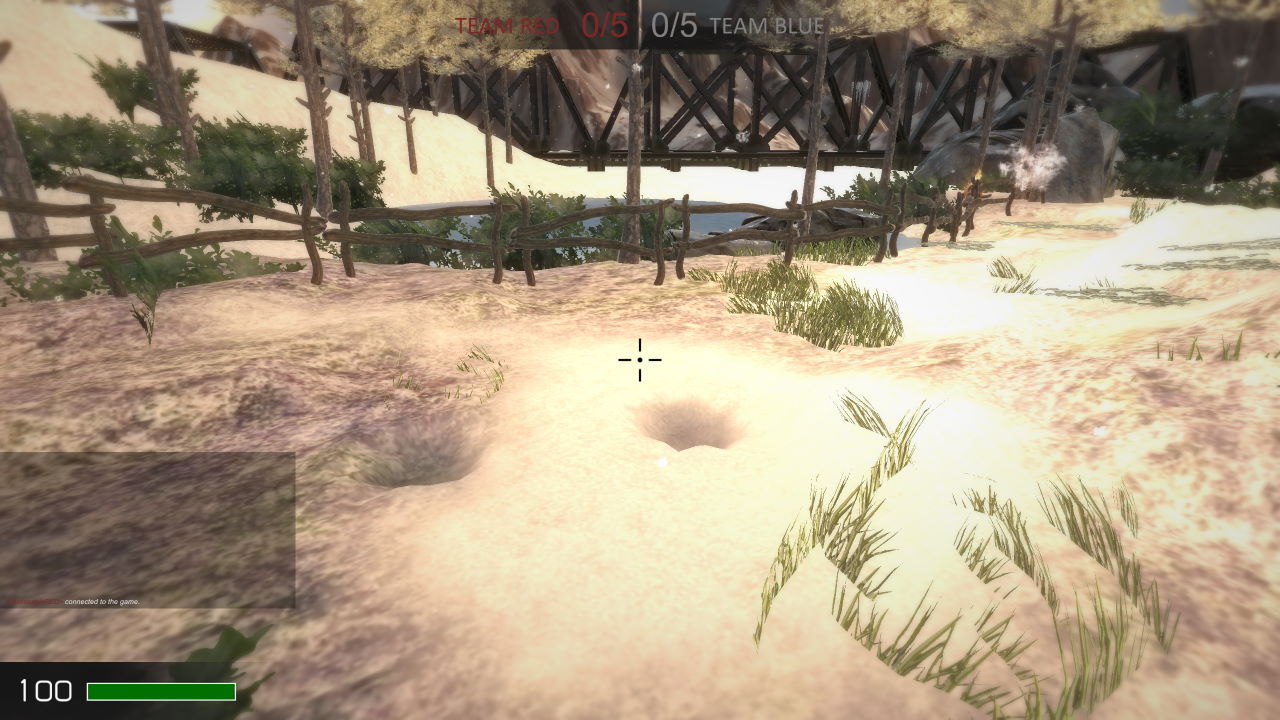